文章内容
一、代理模式分类
- 按照代理创建的时期进行分类,可以分为两类:静态代理、动态代理。
- 静态代理的代理类=原始类+增强(额外功能)+和原始类实现同一个接口,即基于接口的代理。
- 动态代理又分为jdk动态代理,其也是基于接口的代理;cglib的动态代理。
二、动态代理的实现
1、代码实现
实现jdk的动态代理,即基于接口的代理
import lombok.extern.slf4j.Slf4j;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Proxy;
@Slf4j
public class TestProxy {
public static void main(String[] args) {
TestService testService=new TestServiceImpl();
//2. 创建JDK动态代理
InvocationHandler handler = (proxy, method, args1) -> {
System.out.println("------proxy pass before --------");
//原始方法运行
Object ret = method.invoke(testService, args1);
System.out.println("------proxy pass after --------");
return ret;
};
TestService testServiceProxy = (TestService) Proxy.newProxyInstance(TestServiceImpl.class.getClassLoader(), testService.getClass().getInterfaces(), handler);
testServiceProxy.isPass();
}
interface TestService{
boolean isPass();
}
static class TestServiceImpl implements TestService{
@Override
public boolean isPass() {
log.info("测试jdk代理");
//测试代码
return false;
}
}
}
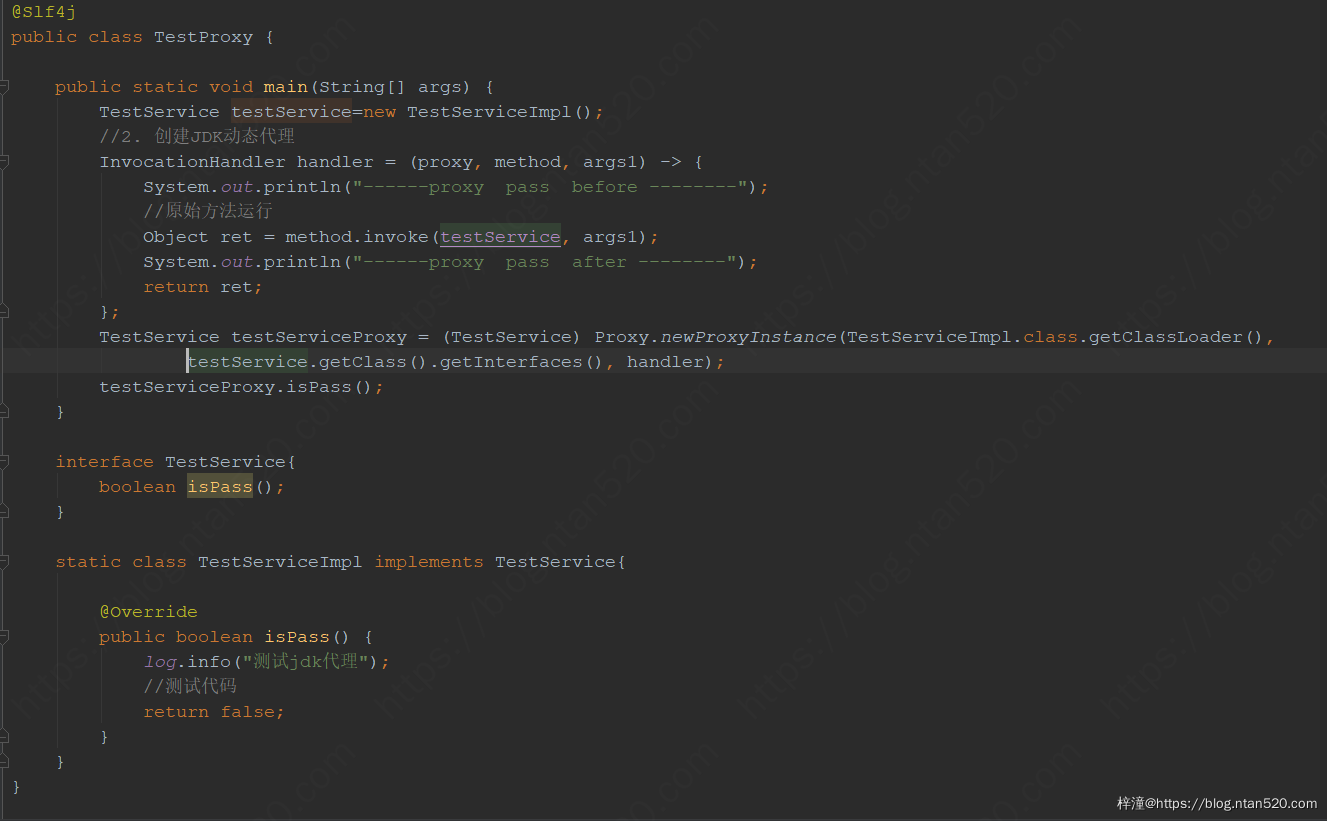
2、输出结果
------proxy pass before --------
19:53:51.279 [main] INFO com.xxx.xxx.controller.TestProxy - 测试jdk代理
------proxy pass after --------
三、实现cglib的动态代理
1、代码实现
import lombok.extern.slf4j.Slf4j;
import org.springframework.cglib.proxy.Enhancer;
import org.springframework.cglib.proxy.MethodInterceptor;
import org.springframework.cglib.proxy.MethodProxy;
import java.lang.reflect.Method;
@Slf4j
public class TestProxy {
public static void main(String[] args) {
AddProxy addProxy=new AddProxy();
TestCglib testCglib=new TestCglib(addProxy);
addProxy= (AddProxy) testCglib.getProxy();
addProxy.test();
}
static class AddProxy{
public void test(){
log.info("..........业务逻辑..........");
}
}
static class TestCglib implements MethodInterceptor {
private Object trarget;
public TestCglib(Object trarget) {
this.trarget = trarget;
}
public Object getProxy() {
Enhancer enhancer = new Enhancer();
enhancer.setSuperclass(trarget.getClass());//目标对象
enhancer.setCallback(this);//
return enhancer.create();
}
@Override
public Object intercept(Object o, Method method, Object[] objects, MethodProxy methodProxy) throws Throwable {
log.info("proxy pass before");
Object object = methodProxy.invoke(trarget, objects);
log.info("proxy pass after");
return object;
}
}
}
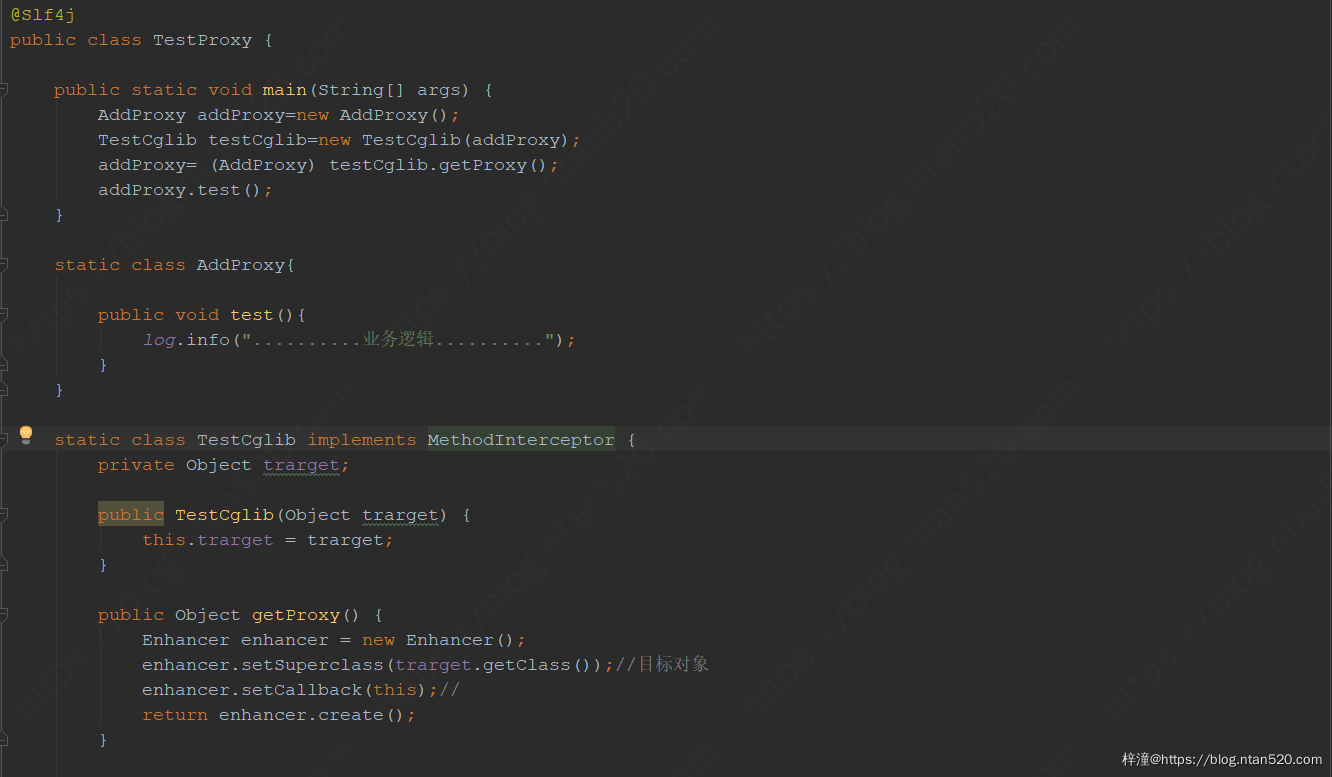
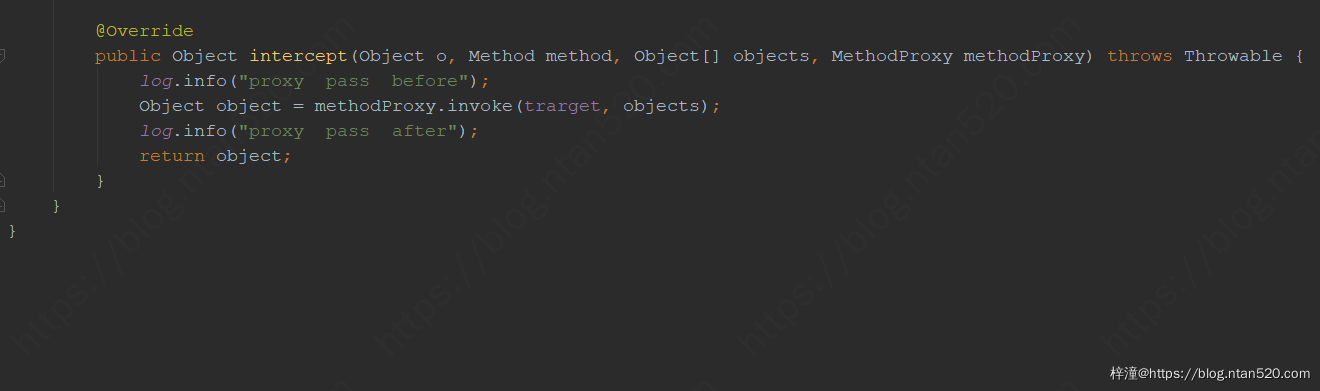
2、输出结果
20:11:26.276 [main] INFO com.xxx.xxx.controller.TestProxy - proxy pass before
20:11:26.306 [main] INFO com.xxx.xxx.controller.TestProxy - ..........业务逻辑..........
20:11:26.306 [main] INFO com.xxx.xxx.controller.TestProxy - proxy pass after