文章内容
在实际开发过程中经常会出现行为不同的实现,比如支付,那可能是微信支付,阿里支付,银联等支付的具体实现。要你用一个设计模式来实现。
一、策略模式定义
策略模式定义了一系列算法,并将每个算法封装起来,使他们可以相互替换,且算法的变化不会影响到使用算法的客户。
二、UML类图
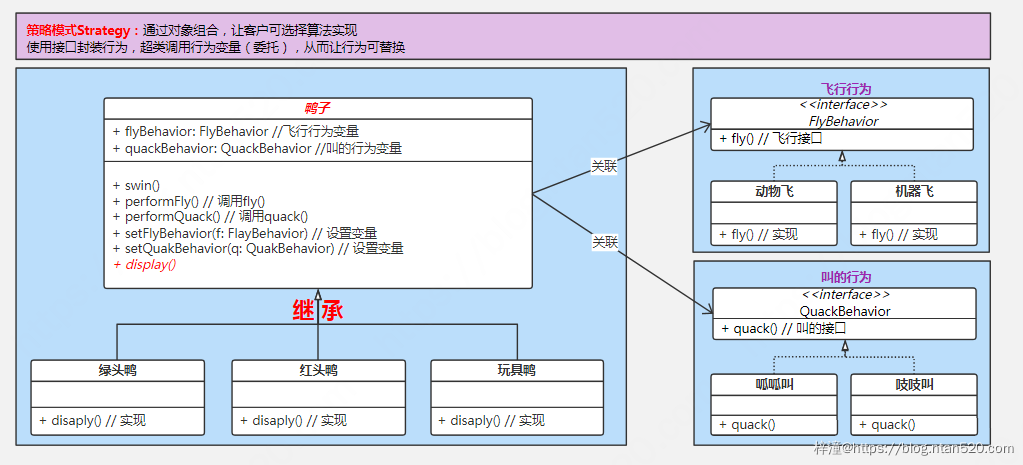
三、具体实现
1、InitializingBean接口说明
- 1. InitializingBean接口为bean提供了初始化方法的方式,它只包括afterPropertiesSet方法,凡是继承该接口的类,在初始化bean的时候都会执行该方法。
- 2. spring初始化bean的时候,如果bean实现了InitializingBean接口,会自动调用afterPropertiesSet方法。
- 3. 在Spring初始化bean的时候,如果该bean实现了InitializingBean接口,并且同时在配置文件中指定了init-method,系统则是先调用afterPropertieSet()方法,然后再调用init-method中指定的方法。
2、策略工厂
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
public class StrategyFactory {
private static final Map<String, PayService> serviceMap = new ConcurrentHashMap<>();
public static void register(PayTypeEnum payTypeEnum, PayService service) {
serviceMap.put(payTypeEnum.getType(), service);
}
public static Boolean getService(String payType) {
PayService payService = serviceMap.get(payType);
if(payService!=null){
return payService.pay(payType);
}
return Boolean.FALSE;
}
}
3、支付枚举
import lombok.AllArgsConstructor;
import lombok.Getter;
@Getter
@AllArgsConstructor
public enum PayTypeEnum {
WX("wx", "微信"),
ZFB("zfb","支付宝支付"),;
private String type;
private String desc;
}
四、具体业务类
1、支付入口
public interface PayService {
Boolean pay(String payType);
}
2、支付入口具体实现
1)微信支付逻辑
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.InitializingBean;
import org.springframework.stereotype.Service;
@Service
@Slf4j
public class WxPayService implements PayService, InitializingBean {
@Override
public void afterPropertiesSet() throws Exception {
StrategyFactory.register(PayTypeEnum.WX,this);
}
@Override
public Boolean pay(String payType) {
log.info("调用微信支付");
return true;
}
}
2)阿里支付具体逻辑
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.InitializingBean;
import org.springframework.stereotype.Service;
@Service
@Slf4j
public class AliPayService implements PayService, InitializingBean {
@Override
public void afterPropertiesSet() {
StrategyFactory.register(PayTypeEnum.ZFB, this);
}
@Override
public Boolean pay(String payType) {
log.info("调用阿里支付");
return true;
}
}
3、定义一个控制器测试
import com.example.demo.celuemoshi.StrategyFactory;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class PayController {
@GetMapping("pay/{type}")
public boolean pay(@PathVariable("type") String type){
StrategyFactory.getService(type);
return true;
}
}
五、测试结果
1、测试微信支付
测试微信支付:http://localhost:10001/pay/wx

2、测试阿里支付
测试阿里支付:http://localhost:10001/pay/zfb
