文章内容
一、前言
现在的开发基本上都是前后端分离,前后端交互都是通过API文档。有了API文档大家各自开发,互不干扰。
API文档主要提供方式:
1、传统方式
传统方式是文档设计好之后,分别发给前端和后端人员。这样有个缺点,接口信息一旦变化,文档就需要重新发送给前后端人员。无法做到实时。所以浪费时间和精力。
2、swagger方式
后台应用集成了swagger之后,会自动暴露出接口,而且这个接口形式还是通过restful风格发布的。一旦后端的接口有变化,会立刻显示出来,因此极大地提高了效率。
swagger方式的好处,那就是后端写的api文档可以通过swagger的形式实时地发布出来,供前端人员查看。
3、其他方式
swagger的页面不好看,也有一些其他的方案,不是有很多bug,就是收费。目前swagger是使用的最多的。
二、swagger集成
前提条件是要新建一个springboot项目。
1、添加依赖
01 02 03 04 05 06 07 08 09 10 | < dependency > < groupId >io.springfox</ groupId > < artifactId >springfox-swagger2</ artifactId > < version >2.9.2</ version > </ dependency > < dependency > < groupId >io.springfox</ groupId > < artifactId >springfox-swagger-ui</ artifactId > < version >2.9.2</ version > </ dependency > |
2.9.2的版本是用的最多的,具体的可以直接去maven的官网去搜索,找一个使用量最多的版本即可。
2、Config配置
新建config包,创建SwaggerConfig类
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 | @EnableSwagger2 @Configuration public class Swagger2Config { @Bean public Docket createRestApi() { return new Docket(DocumentationType.SWAGGER_2) .apiInfo(apiInfo()) .select() //为当前包路径,控制器类包 .apis(RequestHandlerSelectors.basePackage( "com.ntan520.test.controller" )) .paths(PathSelectors.any()) .build(); } //构建 api文档的详细信息函数 private ApiInfo apiInfo() { return new ApiInfoBuilder() //页面标题 .title( "XX平台API接口文档" ) //创建人 "service@ntan520.com" )) //版本号 .version( "1.0" ) //描述 .description( "系统API描述" ) .build(); } } |
这里的配置比较简单。这里有很多选项供去配置。如果项目有多个组,只需要创建多个Docket即可。这时候扫描的包换成每个组的包路径。
3、Controller类中配置
新建一个controller包,然后创建HelloController类
01 02 03 04 05 06 07 08 09 10 11 12 13 14 | @Api ( "Hello控制类" ) @RestController public class HelloController { @GetMapping (value = "/user" ) public User getUser(){ return new User( "Nick Tan" , "123456" ); } @ApiOperation ( "可以指定参数的API" ) @PostMapping ( "/param" ) public String hello2( @ApiParam ( "用户名" ) String name){ return "hello" + name; } } |
这里可以看出,使用注解就可以对这个类、方法、字段等等进行解释说明。其他的字段还有很多,在使用的时候会有相应的提示:
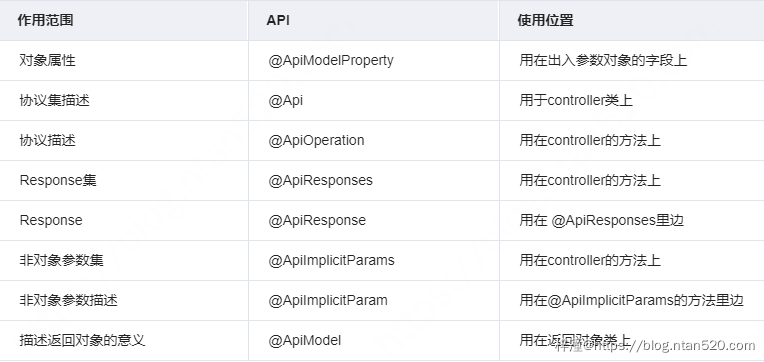
4、查看效果
访问:http://localhost:8080/swagger-ui.html即可

三、常见问题
1、Spring Security – 配置免认证访问
有时候Springboot集成了SpringSecurity,这时候如果访问swagger的地址会自动跳转到登录页面。这是因为SpringSecurity对其进行了拦截。只需要在SpringSecurity配置一下进行放行即可。
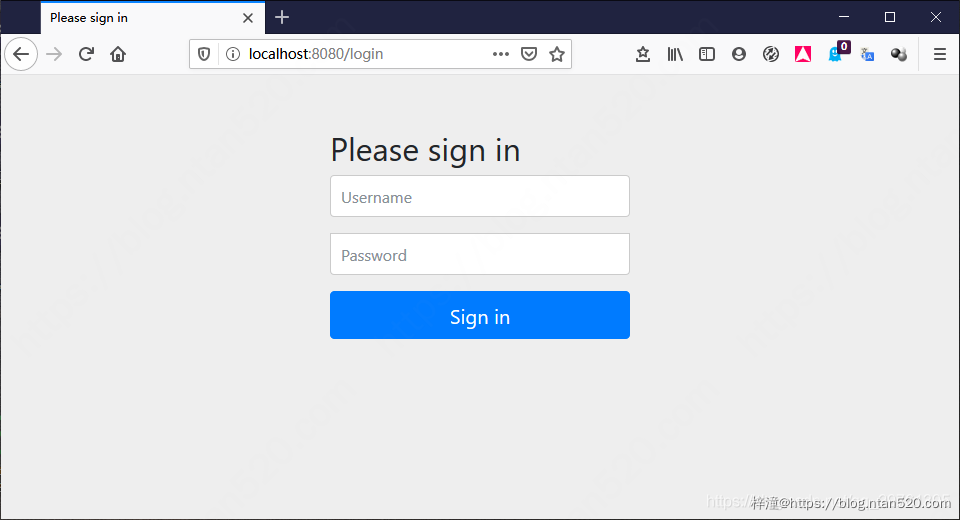
现在配置一下,进行放行。在config包下新建一个SpringSecurityConfig类:
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 | @Configuration @EnableWebSecurity public class SpringSecurityConfig extends WebSecurityConfigurerAdapter { @Override protected void configure(HttpSecurity http) throws Exception { http .authorizeRequests() .antMatchers( "/swagger-ui.html" ).permitAll() .antMatchers( "/webjars/**" ).permitAll() .antMatchers( "/swagger-resources/**" ).permitAll() .antMatchers( "/v2/*" ).permitAll() .antMatchers( "/csrf" ).permitAll() .antMatchers( "/" ).permitAll() .anyRequest().authenticated() .and() .formLogin() ; } } |
此时就可以正常地访问了。
2、为swagger设置jwt
这种方式比较简单,只需要一步即可。修改swaggerConfig类即可。
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 | @EnableSwagger2 @Configuration public class Swagger2Config { @Bean public Docket api() { return new Docket(DocumentationType.SWAGGER_2) .apiInfo(apiInfo()) .securityContexts(Arrays.asList(securityContext())) .securitySchemes(Arrays.asList(apiKey())) .select() .apis(RequestHandlerSelectors.any()) .paths(PathSelectors.any()) .build(); } //构建 api文档的详细信息函数 private ApiInfo apiInfo() { return new ApiInfoBuilder() //页面标题 .title( "XX平台API接口文档" ) //创建人 "service@ntan520.com" )) //版本号 .version( "1.0" ) //描述 .description( "系统API描述" ) .build(); } private ApiKey apiKey() { return new ApiKey( "JWT" , "Authorization" , "header" ); } private SecurityContext securityContext() { return SecurityContext.builder().securityReferences(defaultAuth()).build(); } private List<SecurityReference> defaultAuth() { AuthorizationScope authorizationScope = new AuthorizationScope( "global" , "accessEverything" ); AuthorizationScope[] authorizationScopes = new AuthorizationScope[ 1 ]; authorizationScopes[ 0 ] = authorizationScope; return Arrays.asList( new SecurityReference( "JWT" , authorizationScopes)); } } |
加了一些token验证的代码,比较简单。
3、隐藏Endpoint
有时候Controller,或者是Controller里面的接口方法不想让前端人员看到,可以隐藏即可。
1)隐藏整个Controller
1 2 3 4 5 | @ApiIgnore @RestController public class MyController { //方法 } |
2)隐藏某个接口方法1
1 2 3 4 5 6 | @ApiIgnore @ApiOperation (value = "描述信息" ) @GetMapping ( "/getAuthor" ) public String getAuthor() { return "Nick Tan" ; } |
3)隐藏某个接口方法2
1 2 3 4 5 | @ApiOperation (value = "描述信息" , hidden = true ) @GetMapping ( "/get" ) public LocalDate getDate() { return LocalDate.now(); } |