文章内容
spring中是如何区分每一个bean的?主要是通过以下三种方式:
一、通过XML中的name或者是id属性
1、User类
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 | public class User { private String name; public User(String name) { this .name = name; } public String getName() { return name; } public void setName(String name) { this .name = name; } public void hello() { System.out.println( "name:" + "hello world" ); } } |
2、spring.xml文件,在xml文件中配置bean
01 02 03 04 05 06 07 08 09 10 | <?xml version= "1.0" encoding= "UTF-8" ?> <beans xmlns= "http://www.springframework.org/schema/beans" xsi:schemaLocation="http: //www.springframework.org/schema/beans http: //www.springframework.org/schema/beans/spring-beans.xsd"> <bean id= "user" class = "com.example.demo.bean.User" > <constructor-arg value= "愚公要移山" /> </bean> </beans> |
在resource目录下面新建了一个META-INF目录,用于存放spring.xml文件。这个文件中使用了id来区分不同的bean。
3、测试
01 02 03 04 05 06 07 08 09 10 | public class Main { public static void main(String[] args) { String xmlPath = "META-INF/spring.xml" ; ApplicationContext applicationContext = new ClassPathXmlApplicationContext(xmlPath); //从spring容器获得 User user = (User) applicationContext.getBean( "user" ); user.hello(); } } |
4、运行结果
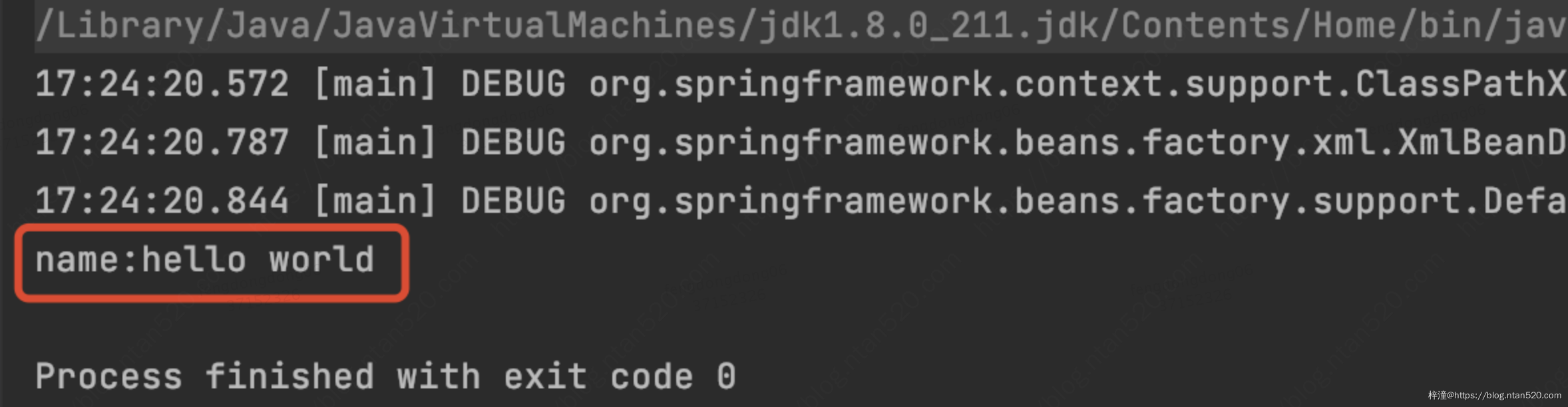
二、通过注解的方式
1、声明Bean的注解
- @Component组件,没有明确的角色。
- @Service在业务逻辑层(Service层)使用。
- @Repository在数据访问层(dao层)使用。
- @Controller在展现层使用。
它们默认没有直接指定bean的name,所以bean的name是spring自动生成的。bean的name生成规则如下:
- class的simpleName如果大于1个字符且第二个字符是大写字母,则simpleName就是bean name
- 如果class的simpleName是1个字符或者第2个字符是小写,则将首字母转为小写的字符串作为bean name
举例 “FooBah”的bean名称变成了”fooBah”,”X” 变成了 “x”,”URL” 依然是”URL”。
注意:
若不同的包下有两个名字相同的类,而这两个类都声明成spring的bean,这时候就会产成冲突。因为bean的名字就是bean的唯一标示,是不允许重复的。
2、UserService
1 2 3 4 5 6 | public class UserService { public void hello() { System.out.println("hello world"); } } |
3、config配置
1 2 3 4 | @Configuration @ComponentScan ( "com.example.demo.bean" ) public class JavaConfig { } |
4、测试
1 2 3 4 5 6 7 8 9 | public class Main { public static void main(String[] args) { AnnotationConfigApplicationContext context = new AnnotationConfigApplicationContext(JavaConfig. class ); UserService teacherService = (UserService) context.getBean( "userService" ); teacherService.hello(); context.close(); } } |
5、运行结果
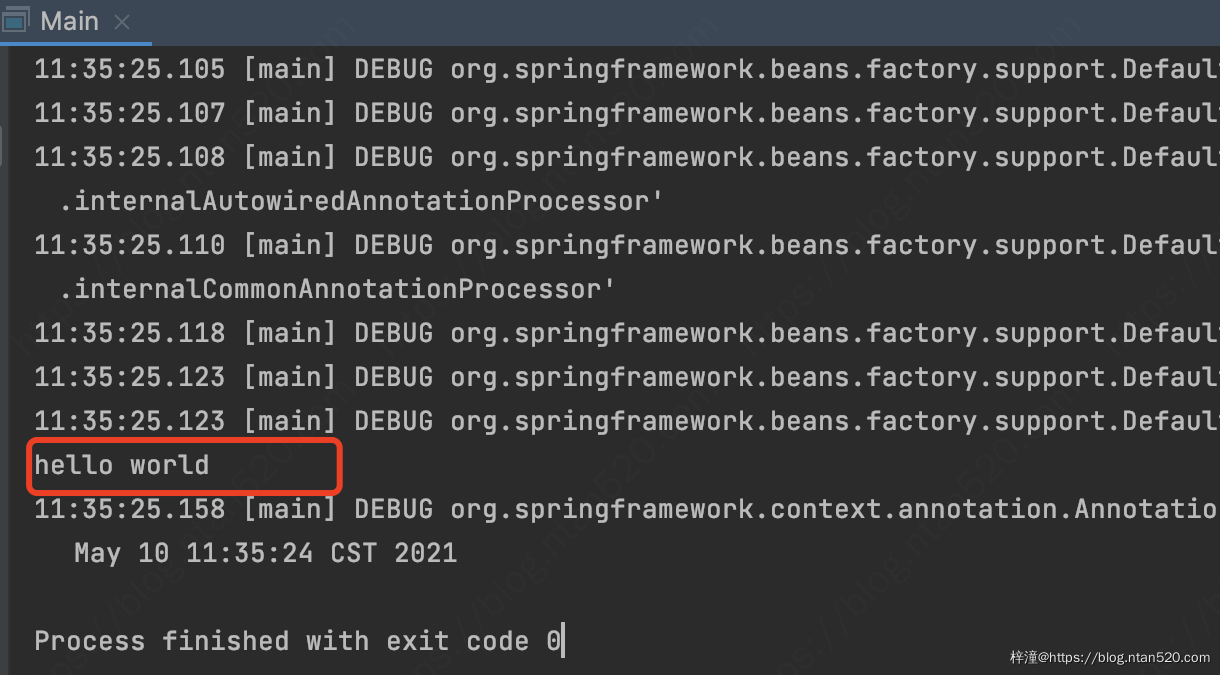
三、通过Java配置
Java配置是通过@Configuration和@Bean来实现的。
- @Configuration声明当前类是一个配置类,相当于一个Spring配置的xml文件。
- @Bean注解在方法上,声明当前方法的返回值为一个Bean。
- @Bean 注解声明的方法名是放入spring容器的bean的name。
1、JavaConfig
01 02 03 04 05 06 07 08 09 10 | @Configuration @ComponentScan ( "com.example.demo.bean" ) public class JavaConfig { @Bean public UserService getStudentService() { UserService userService = new UserService(); return userService; } } |
此时的bean注解到了方法上,根据上面的定义,此时的bean是返回类型UserService,因此返回的结果也是。
2、测试
1 2 3 4 5 6 7 8 9 | public class Main { public static void main(String[] args) { AnnotationConfigApplicationContext context = new AnnotationConfigApplicationContext(JavaConfig. class ); UserService teacherService = (UserService) context.getBean( "userService" ); teacherService.hello(); context.close(); } } |
3、运行结果
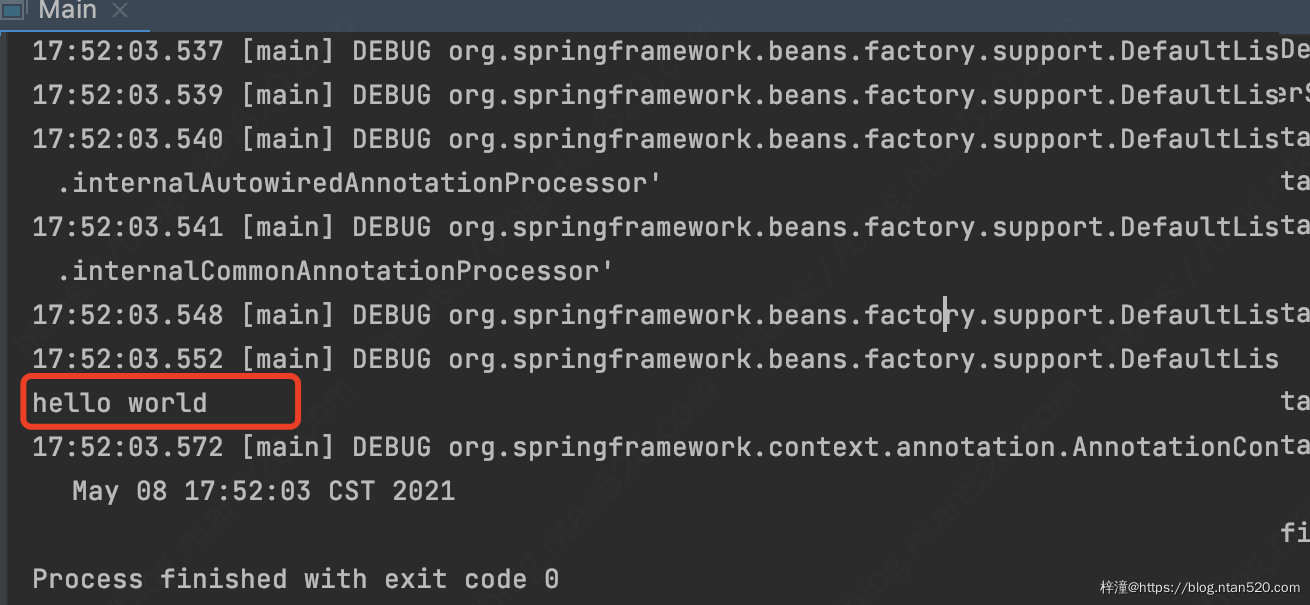