文章内容
Spring中提供了JavaMailSender接口实现邮件发送功能,在SpringBoot2.X中也封装了发送邮件相关的Starter,并且提供了自动化配置。
一、添加对应的Starter
pom.xml中添加下面依赖:
1 2 3 4 | < dependency > < groupId >org.springframework.boot</ groupId > < artifactId >spring-boot-starter-mail</ artifactId > </ dependency > |
二、添加发送邮件相关的配置
application.properties中添加邮件配置:
1 2 3 4 5 6 7 8 9 | spring.mail.host = smtp.163.com spring.mail.port = 465 spring.mail.username = xxx@163.com spring.mail.password = xxx spring.mail.properties.mail.smtp.auth = true spring.mail.properties.mail.smtp.timeout = 25000 spring.mail.properties.mail.smtp.socketFactory.class = javax.net.ssl.SSLSocketFactory spring.mail.properties.mail.smtp.starttls.enable = true spring.mail.properties.mail.smtp.starttls.required = true |
三、实现发送邮件功能
本例中封装了一个MailServiceImpl,里面注入javaMailSender即可,具体代码如下:
1、MailServiceImpl.java部分代码
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | /** * 发送邮件 */ public void sendMail(Email email) { long start = System.currentTimeMillis(); try { MimeMessage mimeMessage = javaMailSender.createMimeMessage(); //true表示需要创建一个multipart message MimeMessageHelper helper = new MimeMessageHelper(mimeMessage, true , "UTF-8" ); helper.setFrom(mailUserName); helper.setTo(email.getToAddress().toArray( new String[email.getToAddress().size()])); helper.setSubject(email.getSubject()); helper.setText(email.getContent(), true ); if ( null != email.getAttachments() && email.getAttachments().size() > 0 ) { for (File curFile : email.getAttachments()) { FileSystemResource file = new FileSystemResource(curFile); helper.addAttachment(MimeUtility.encodeWord(file.getFilename(), "utf-8" , "B" ), file); } } log.info( "邮件开始发送" ); javaMailSender.send(mimeMessage); long sendMillTimes = System.currentTimeMillis() - start; log.info( "邮件发送成功,sendTimes=" + sendMillTimes); } catch (Exception e) { log.error( "发送html邮件时发生异常!" , e); } } |
2、MailController.java部分代码
01 02 03 04 05 06 07 08 09 10 11 12 13 | @RequestMapping (value = "/sendMail" ) public String sendEmail() { Email email_email = new Email(); List<String> addressList = new ArrayList<String>(); addressList.add( "xxx@qq.com" ); email_email.setToAddress(addressList); email_email.setSubject( "java-主题测试" ); // 主题 email_email.setContent( "你好!<br><br> 测试邮件发送成功!" ); // 发送邮件 mailService.sendMail(email_email); return "ok" ; } |
3、运行效果
项目启动成功后访问http://localhost:8080/sendMail即可成功发送邮件,收到邮件截图如下:
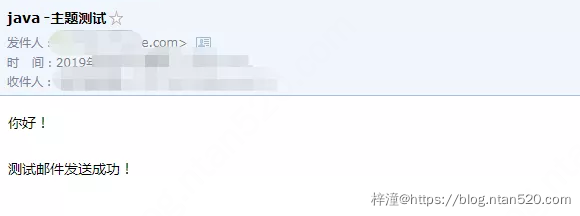
四、实现过程中遇到的问题
1、中文附件乱码问题
java mail发邮件是附件名过长默认会被截断显示乱码,在程序启动时设置主动设置为false可正常显示,具体设置代码如下:
1 2 3 4 5 | public static void main(String[] args) { //java mail发邮件时附件名过长默认会被截断,附件名显示【tcmime.29121.29517.50430.bin】,主动设为false可正常显示附件名 System.setProperty( "mail.mime.splitlongparameters" , "false" ); SpringApplication.run(SendMailApplication. class , args); } |
2、无法注入JavaMailSender问题
在application.properties中配置spring.mail.host即可。